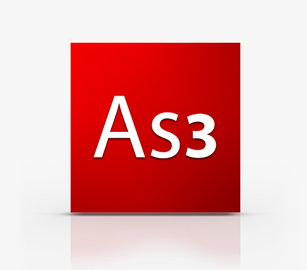
Learning ActionScript (Part 1)
ActionScript Fundamentals
This is section 1 of the ActionScript training notes I wrote while Multimedia Coordinator for Box Hill Institute.
1.0 Introduction
ActionScript is a developer-level scripting language that lets you add interactivity to your animations by tying a graphic element (e.g. a button) to a specific action (e.g. opening up a specific webpage).
ActionScript and JavaScript have a lot in common. They’re both scripting languages, meaning that they are programming languages that run inside of other programs, ActionScript runs inside of Flash or Flex, and JavaScript runs inside HTML. They both are derived from the same programming specification ECMA-262.
ActionScript 3.0 was released with Adobe Flex 2.0 and Flash CS3. ActionScript 1 and 2 are still supported by Flash CS3 but you cannot mix ActionScript 3.0 code with that of earlier versions. This is because the Flash Player uses two completely separate virtual machines to interpret the code from 1/2 and 3. The new virtual machine for 3.0 runs the code much faster than the earlier versions.
1.1 Exploring the Actions Panel
You use the Actions panel to build the ActionScript code that turns regular animations into interactive animations like clickable splash pages, navigation bars, and type-in forms.
The Actions panel lets you mix and match snippets of ActionScript code to build what’s called an ActionScript “script”, which you can then attach to one of the “frames” in your animation to make your animation “smart.“ After you create a script, the ActionScript debug panels (e.g. the Output panel shows errors and output from trace() instructions) let you troubleshoot any scripting code that’s giving you trouble.
Ask more than one script writer where it’s best to place code—Flash timeline or ActionScript file—and you’re likely to start an energetic debate. If you plan on a long Flash career, it’s worth learning both techniques.
1.1.1 Timeline Programming
Timeline programming is the way everyone used to write ActionScript. You attach scripts to individual frame in the Flash timeline. Quick and easy, this method gives a certain amount of instant gratification. If you quickly want to test an idea, the tendency is to attach some code to the timeline.
The problem is that you may end up with snippets of ActionScript code in many places, which makes it more difficult to troubleshoot the code if something goes wrong. It’s even worse if you (or someone else) return to a project years later to make some changes.
1.1.2 File Programming
ActionScript file prgramming is the preferred method for large projects and true object-oriented programming. One of the goals of object-oriented programming is to create code that’s easily reusable.
- The code has to be readable and understandable.
- The chunks of code have to be somewhat independent.
Placing all your code in a seperate .as file forces you to provide more thorough definitions of the objects in your FLash project. As a result you write more lines of code, but there is a better chance to reuse that code for other projects. When teams of programmers work simultaneously on the same project, it’s much easier to update the code and keep track of each updated version. Artists can work on the drawing aspect of the .fla file and programmers can write the controlling code in seperate .as files.
1.2 Learning basic ActionScript syntax
1.2.1 Debugging:
trace("This is a test. i=" + i); //view debugging messages and values to output panel
1.2.2 Timeline control:
stop(); //stop playing at current frame
gotoAndStop(frameNum); //jump to frameNum on the current timeline and pause there
gotoAndPlay(frameNum); //jump to frameNum on the current timeline and play from there
1.2.3 MovieClip control:
demo_mc.stop(); //pause playing the demo_mc MovieClip only
demo_mc.play(); //continue playing the demo_mc MovieClip only
demo_mc.visible = false; //hide the movieClip
1.3 Creating variables
i = 0; //shorthand, dynamic type
var i:Number = 0; //variable type defined and initialised
_root.i = 6; //old Actionscript 2.0 way of referring to variable on stage
(MovieClip)(this.parent).i = 6; //Actionscript 3.0 equiv.
1.3.1 Naming conventions:
var thisIsAVariableName:Number = 1; //standard variable in CamelCase
var thisIsAMovieClip_mc:MovieClip = new MovieClip(); //MovieClip object with _mc at end
const THIS_IS_A_CONSTANT:String = "test; //Constant all in UPPERCASE
1.3.2 Variable types:
var text:String = "this is a test"; //String contain alphanumerics
var i:Number = 27.536; //Number contains any number
var isOn:Boolean = true; //Boolean contains true or false (for comparing)
var i:int = -16; //int contains whole positive & negative numbers
var i:uint = 16; //uint contains only whole positive numbers
var myClip_mc:MovieClip = new MovieClip(); //MovieClips, Buttons, Sounds etc in this format
var list:Array = [“word1”, “word2”, “word3”, “word4”]; //Array is a list of any values
var Object = {x:20, y:15, z:-30}; //Object is a powerful & versatile way of grouping values
1.3.3 Assigning values:
var text:String = "this is a test"; //basic assigning a value to a variable
text = "this is a test" + " as well"; //String concatenation or joining
var i:Number = 0; //initialise a new variable
i = i + 1; //increment an existing variable by 1
i++; //shorthand for incrementing an existing variable by 1
i--; //shorthand for decrementing an existing variable by 1
i+=2; //shorthand for incrementing an existing variable by 2
1.4 Creating loops
1.4.1 while loop
var index:uint = 0;
while (index<10) {
trace(“index = “ + index);
}
1.4.2 for loop
var list:Array = [“word1”, “word2”, “word3”, “word4”];
for (int i:int = 0; i<list.length(); i++) {
trace(“item “ + i + “ = ” + list[i]);
}
will output on the screen:
item 0 = word1
item 1 = word2
item 2 = word3
item 3 = word4
1.4.3 for .. each .. in loop
The for each..in loop iterates through the items of a collection, which can be tags in an XML or XMLList object, the values held by object properties, or the elements of an array. For example, as the following excerpt shows, you can use a for each..in loop to iterate through the properties of a generic object, but unlike the for..in loop, the iterator variable in a for each..in loop contains the value held by the property instead of the name of the property:
var myObj:Object = {x:20, y:30};
for each (var num in myObj) {
trace(num);
}
// output:
// 20
// 30
You can iterate through an XML or XMLList object, as the following example shows:
var myXML:XML =
Jane
Susan
John
;
for each (var item in myXML.fname) {
trace(item);
}
/* output
Jane
Susan
John
*/
You can also iterate through the elements of an array, as this example shows:
var myArray:Array = [“one”, “two”, “three”];
for each (var item in myArray) {
trace(item);
}
// output:
// one
// two
// three
You cannot iterate through the properties of an object if the object is an instance of a sealed class, even for instances of dynamic classes.
1.5 Using conditional logic
1.5.1 Boolean logic:
- Comparison operators:
- , ==, =, !=
- Boolean operators:
- &&, ||
Example:
if (i>0 && i<10) {
trace(“i is greater than 0 and smaller than 10”);
} else {
trace(“i is smaller than or equal to 0 or greater than or equal to 10”);
}
1.5.2 if else statement
- Is used for branching at a decision point depending on value
- Remember to always include an else case, even if you put a // or ; as a marker
if (i>0) {
trace("i is greater than 0");
} else {
trace("i is smaller than or equal to 0");
}
1.5.2 Compound if else statement
- Is used for multiple branching at a decision point depending on value
if (i>0) {
trace(“i is greater than 0”);
} else if (i>-10) {
trace(“i is smaller than or equal to 0 and greater than -10”);
} else {
trace(“i is smaller than or equal to -10”);
}
1.5.3 switch statement
- The most efficient way of multiple branching at a decision point depending on value
switch (i) { //i is the variable being tested
case 1 : //if (i=1) then ...
trace("i = 1");
break; //need to include to exit out of switch here
case 2 : //if (i=2) then ...
trace(“i = 2”);
break;
case 3 : //if (i=3) then ...
trace(“i = 3”);
break;
default : //else ...
trace(“i is any other value except 1, 2, and 3”);
break;
}
1.6 Creating functions
Definition:
function functionName(parameter1:type, parameter2:type, ...) :returntype {
operation1;
...
operationn;
}
Example 1:
function test() :void {
trace("function test was called");
}
test();
Example 2:
function test2(value1:Number, value2:String) :String {
var output:String = null;
if (value1>50 && value2=="years") {
output = "He is old";
} else {
output = “He is young”; }
return output;
}
trace("the result of test2 = " + test2(60, "years"));
1.7 Including external ActionScript files
External ActionScript files must written in a package/class structure. Packages parallel the folder structure where the ActionScript files are stored. Classes define object templates that can be instantiated and used within your Flash document. These Object instances are similar to MovieClips in usage within Flash. Objects can contain their own public visible variables and functions that may be called by Flash. This will be elaborated more in Section 2.
There are two ways of using external ActionScript files from within your Flash file:
- Automatically included .as file
- Enter .as filename within the text field on the “properties” panel in Flash.
- Imported .as files
- type an import statement within the Actions panel:
import packageName.ClassName; //imports a particular class from the package folder
import packageName.*; //imports all classes from the package if required
- type an import statement within the Actions panel:
1.8 Handling exceptions
As you start to write your own ActionScript routines and publish to SWFs you will notice various error messages turn up on your Output panel. There are various types of errors:
- Compile-time errors occur if you have typed things in incorrectly or not defined commands or objects correctly in your code. The best thing is to review your code and look at the Flash help files for further information. It is best to fix your errors one at a time, as listed in the Output window.
- Run-time errors occur when you try to load external files that don’t exist, or work with dynamic data that is different to what you code expects. These are harder to fix. The best approach is to take things step-by-step, turning some problem code off temporarily using comments (// or /* */), and inserting trace() statements around the problem areas of code. Once you find out where the problem is, look up the help and try some ActionScript forums on the web to help you understand.