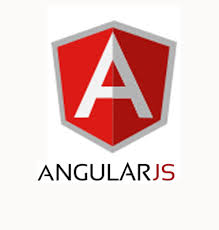
AngularJS Framework
HTML is great for declaring static documents, but it falters when we try to use it for declaring dynamic views in web-applications. AngularJS by Google is a JavaScript MVC framework that lets you extend HTML vocabulary for your application and lets you build well structured, easily testable, and maintainable front-end applications.
The framework consists of a tightly integrated toolset that will help you build well structured, rich client-side applications in a modular fashion—with less code and more flexibility. AngularJS extends HTML by providing directives that add functionality to your markup and allow you to create powerful dynamic templates. You can also create your own directives, crafting reusable components that fill your needs and abstracting away all the DOM manipulation logic.
It also implements two-way data binding, connecting your HTML (views) to your JavaScript objects (models) seamlessly. In simple terms, this means that any update on your model will be immediately reflected in your view without the need for any DOM manipulation or event handling (e.g., with jQuery).
Angular provides services on top of XHR that dramatically simplify your code and allow you to abstract API calls into reusable services. With that, you can move your model and business logic to the front-end and build back-end agnostic web apps.
Angular is very flexible regarding server communication. Like most JavaScript MVC frameworks, it lets you work with any server-side technology as long as it can serve your app through a RESTful web API. But Angular also provides services on top of XHR that dramatically simplify your code and allow you to abstract API calls into reusable services. As a result, you can move your model and business logic to the front-end and build back-end agnostic web apps.
The following is a very basic example of how to use AngularJS in your HTML.
Example Source:
<div ng-app="">
<p>Input something in the input box:</p>
<p>Name: <input type="text" ng-model="name" value="John"></p>
<p ng-bind="name"></p>
<div>name = {{name}}</div>
</div>
<script src="//ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script>
Example Live:
Input something in the input box:
Name:
Angular-Seed
To start any any app it is best to start with some boilerplate. I recommend the angular-seed project as it not only provides you with a great skeleton for bootstrapping, but also sets the ground for unit testing with Karma and Jasmine.
Angular-Seed is an application skeleton for a typical AngularJS web app. You can use it to quickly bootstrap your angular webapp projects and dev environment for these projects.
The seed contains a sample AngularJS application and is preconfigured to install the Angular framework and a bunch of development and testing tools for instant web development gratification.
The seed app doesn’t do much, just shows how to wire two controllers and views together.
Getting Started
To get you started you can simply clone the angular-seed repository and install the dependencies:
Prerequisites
You need git to clone the angular-seed repository. You can get it from http://git-scm.com/.
We also use a number of node.js tools to initialize and test angular-seed. You must have node.js and its package manager (npm) installed. You can get them from http://nodejs.org/.
Clone angular-seed
Clone the angular-seed repository using git:
git clone https://github.com/angular/angular-seed.git
cd angular-seed
Install Dependencies
We have two kinds of dependencies in this project: tools and angular framework code. The tools help us manage and test the application.
We get the tools we depend upon via npm, the node package manager.
We get the angular code via bower, a client-side code package manager.
We have preconfigured npm to automatically run bower so we can simply do:
npm install
Behind the scenes this will also call bower install. You should find that you have two new folders in your project.
node_modules - contains the npm packages for the tools we need
app/bower_components - contains the angular framework files
Note that the bower_components folder would normally be installed in the root folder but angular-seed changes this location through the .bowerrc file. Putting it in the app folder makes it easier to serve the files by a webserver.
Run the Application
We have preconfigured the project with a simple development web server. The simplest way to start this server is:
npm start
Now browse to the app at: http://localhost:8000/app/index.html.
Directory Layout
app/ --> all of the files to be used in production
css/ --> css files
app.css --> default stylesheet
img/ --> image files
index.html --> app layout file (the main html template file of the app)
index-async.html --> just like index.html, but loads js files asynchronously
js/ --> javascript files
app.js --> application
controllers.js --> application controllers
directives.js --> application directives
filters.js --> custom angular filters
services.js --> custom angular services
partials/ --> angular view partials (partial html templates)
partial1.html
partial2.html
test/ --> test config and source files
protractor-conf.js --> config file for running e2e tests with Protractor
e2e/ --> end-to-end specs
scenarios.js
karma.conf.js --> config file for running unit tests with Karma
unit/ --> unit level specs/tests
controllersSpec.js --> specs for controllers
directivessSpec.js --> specs for directives
filtersSpec.js --> specs for filters
servicesSpec.js --> specs for services