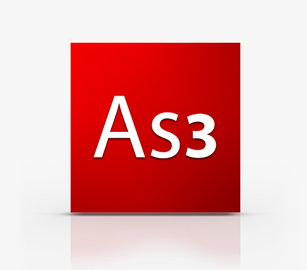
Learning ActionScript (Part 3)
3.0 Using Button and MovieClip objects
3.1 Scope – this & parent
Although a bit nebulous for some just starting with ActionScript, this can be your friend. It is essentially shorthand for “whichever object or scope you’re working with now.” Scope is the realm or space within which an object lives. For example, think of a movie clip inside Flash’s main timeline. Each of these objects has a unique scope, so a variable or function defined inside the movie clip will not exist in the main timeline, and vice versa.
It is easiest to understand the usage of this in context, but here are a couple of examples to get you started. If you wanted to refer to the width of a nested movie clip called mc from within the main timeline, you might say:
this.mc.width;
If you wanted to refer to the main timeline from the nested movie clip, you might write:
this.parent.mc.width;
In both cases, this is a reference point from which you start your path. It is fairly common to drop the keyword when going down from the current scope (as in the first example), but it is required when going up to a higher scope (as in the second example). This is because Flash must understand what the parent is actually a parent of in order to start traversing through the hierarchy.
Imagine a family reunion in which several extended family members, including cousins and multiple generations, are present, and you are looking for your mother, father, or grandparent. If you just said “parent,” any number of parents might answer. If you, instead, said “my parent” or “my mother’s parent,” that would be specific enough to get you headed in the right direction.
3.2 Referencing objects dynamically from a String using “this[]”
When dealing with a large number of objects such as buttons and movieclips, you can create a dynamic runtime reference to these objects from a string:
var temp_mc:Object = null;
for (var i:int=0; i<100; i++) {
temp_mc = this[“test“ + index + “_mc“];
if (temp_mc!=null) {
temp_mc.visible = false;
}
}
trace(this[“content_mc”][“level2_mc”][“course_txt”][“htmlText”]); //this accesses the dot structure below
trace(content_mc.level2_mc.course_txt.htmlText]);
3.3 MovieClips as Buttons
Any MovieClip can act as a SimpleButton with the addition of the buttonMode and mouseChildren parameters specified in your Actionscript code.
clip_mc.buttonMode = true; //show a hand and button states when rolled over
clip_mc.mouseChildren = false; //stop mouse rollingoff on text etc inside the clip_mc
clip_mc.mouseEnabled = true; //enable a MovieClip to be visible to the mouse
function demoMouseRolledOver(evt:MouseEvent) :void {
if (evt!=null && evt.target!=null) {
trace(“demoMouseRolledOver called with “ + evt.target.name);
switch (evt.target.name) {
case “clip_mc“ :
//do stuff
break;
case “other_mc“ :
//do stuff
break;
default :
//all other buttons caught here
break;
}
}
}
clip_mc.addEventListener(MouseEvent.MOUSE_OVER, demoMouseRolledOver);
clip_mc.addEventListener(MouseEvent.MOUSE_OUT, demoMouseRolledOff);
clip_mc.addEventListener(MouseEvent.MOUSE_UP, demoMouseClicked);
clip_mc.addEventListener(MouseEvent.MOUSE_DOWN, demoMouseDragging);